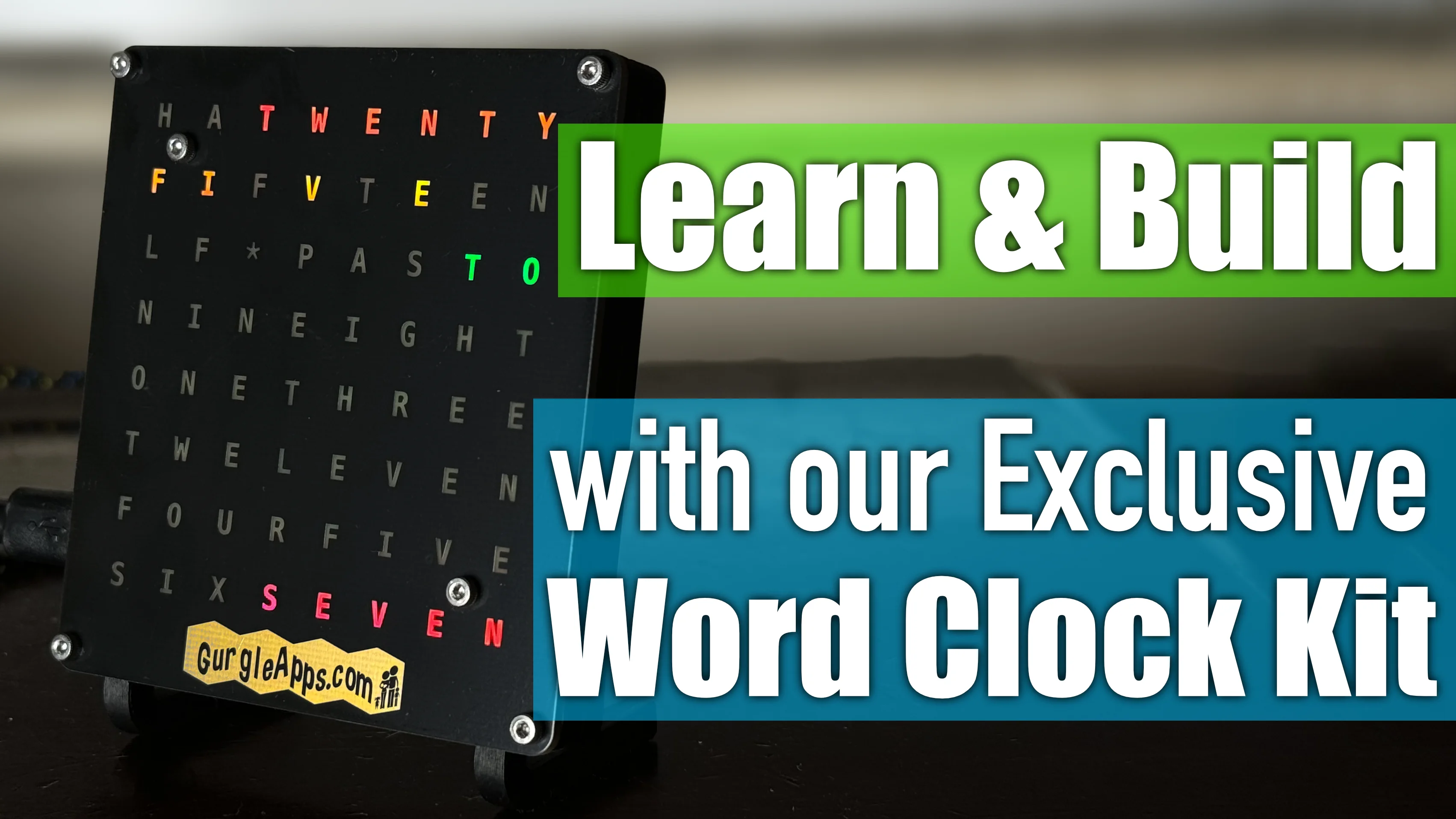
MicroPython & ArduPy On Wio Terminal
- Overview
- Video
- How to get into bootloader mode
- Installing the MicroPython firmware
- Flashing the firmware to the Wio Terminal
- Installing aip
- Using aip for repl
- The code flashing the 2 LEDs
Overview
We will show you how to get MicroPython, ArduPy, and aip on the Wio Terminal. aip is optional but if you wanted to connect to your Wio Terminal through REPL in the shell or Thonny you will need aip. We also have a tutorial on CircuitPython for the Wio Terminal if you prefer to use CircuitPython.
Video
How to get into bootloader mode
This is shown 48 secs into the video but in short you just plug the Wio Terminal into your computer then simply flick the switch (on the side of the Wio Terminal) all the way away from the off side and back to the middle 2x really quickly- I mean really fast! It should pop up as a Mass Storage device on your computer.
Installing the MicroPython firmware
To download the firmware, click the link below:
MicroPython firmare for wio
Flashing the firmware to the Wio Terminal
Once the firmware has downloaded, drag or copy the .UF2 file to your Mass Storage device. Now, your board will disappear from your computer. It will then appear back on your computer(if it doesn't you may have to reset the Wio Terminal).
It will name itself ARDUPY but if it doesn't it still has worked. Also, any old files you had on the Wio Terminal will still be on it. There should be a file called main.py - this is the file you edit and whenever you save that file the code in that file will be executed. If it doesn't have the file main.py you can just make a new file and call it main.py.
If you want your code to run when you reset your MicroPython device or turn it on you save your code as boot.py .
The code below will blink the onboard LED:
from machine import Pin, Map
import time
LED = Pin(Map.LED_BUILTIN, Pin.OUT)
while True:
LED.on()
time.sleep(1)
LED.off()
time.sleep(1)
Installing aip
For this you need pip3 - if you don't have this just type the following into your shell:
sudo apt update
sudo apt install python3-pip
Now you have pip3!
next to get aip we need to type:
pip3 install ardupy-aip
running aip straight off
aip
came up with an error for me. If there was no error for you skip this part. To fix the error you would need to add the path to aip to your PATH environment variable. For speed we just used cd to change directory to where aip was stored and ran it from there.
cd ~/.local/bin
./aip
Using aip for repl
repl is really helpful for testing things out since you can run MicroPython code straight onto the Wio Terminal. To get into repl you type:
aip shell -c "repl"
If you had to change directory to where aip is stored you would type:
./aip shell -c "repl"
You can now have a play around with repl.
The code flashing the 2 LEDs
The wio terminal has stickers to stick next to the pins so you can use those numbers on the sticker as your GPIO Pins. If you use different pins to us just change the ORANGE_LED = 3
or BLUE_LED = 5
to your Pin of choice.
from machine import Pin, Map
import time
ORANGE_LED = 3
BLUE_LED = 5
LED = Pin(BLUE_LED, Pin.OUT)
while True:
LED.on()
time.sleep(1)
LED.off()
time.sleep(1)
Related Posts
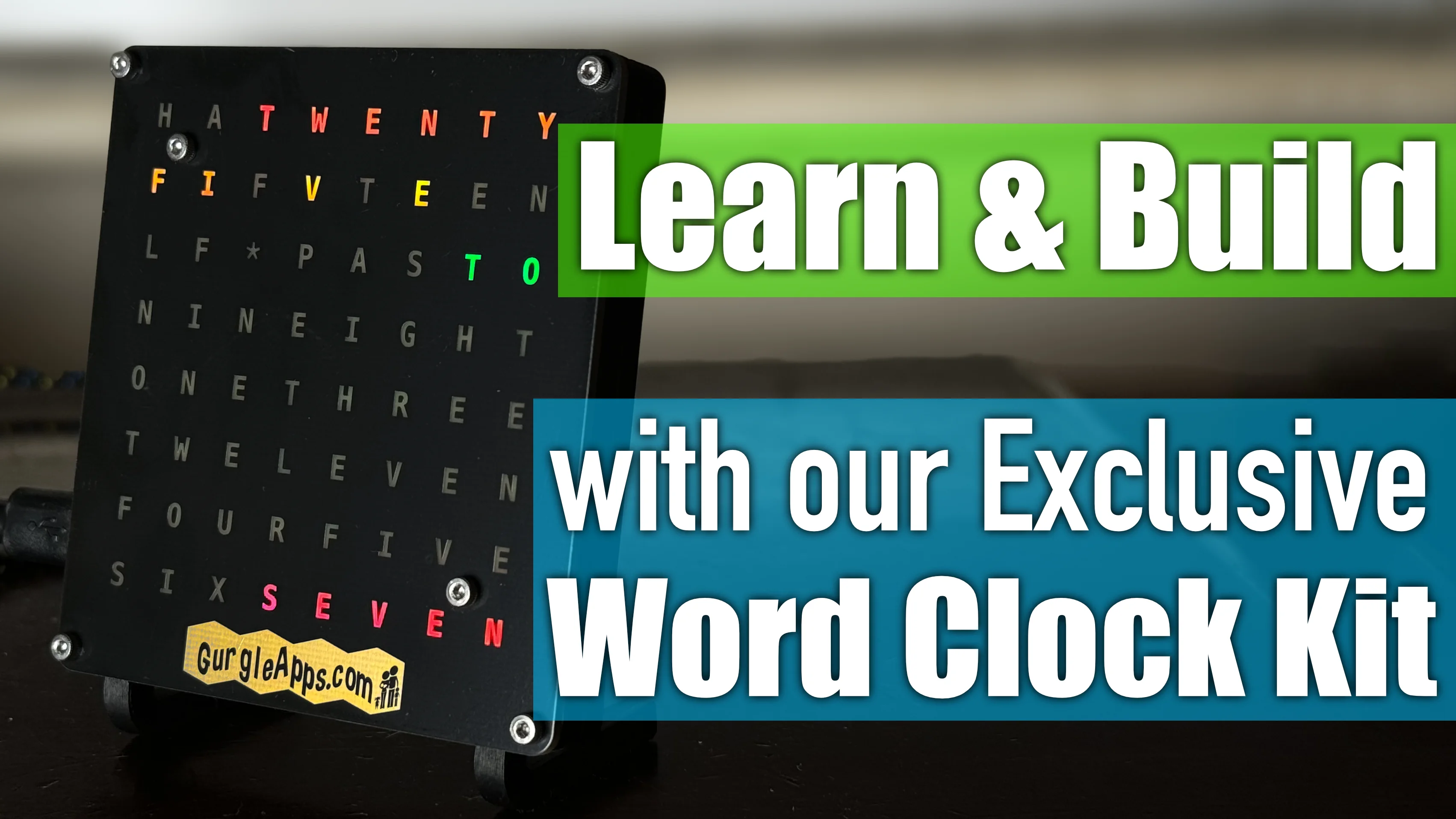
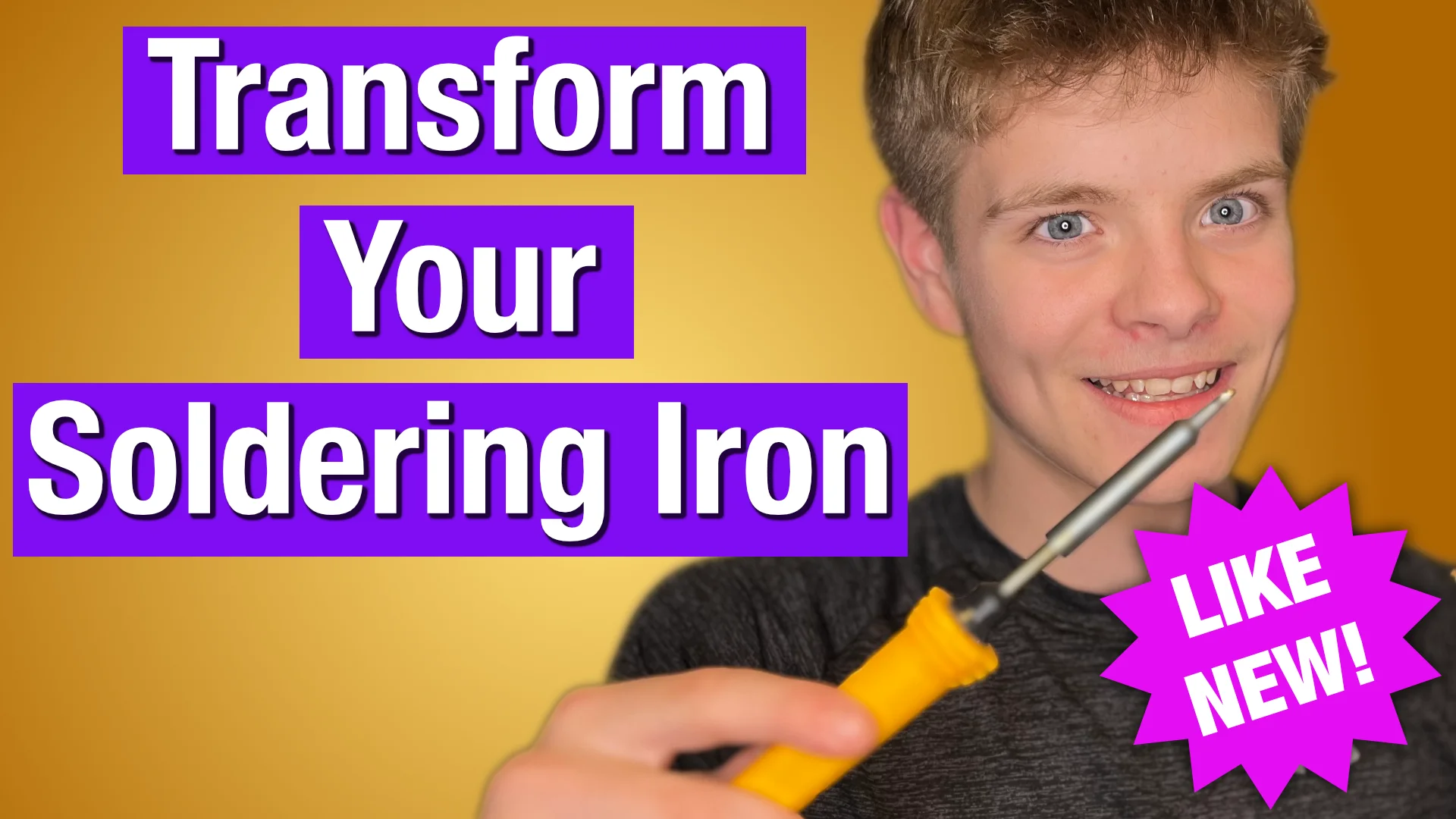
Transform Your Soldering Iron With Tip Tinner
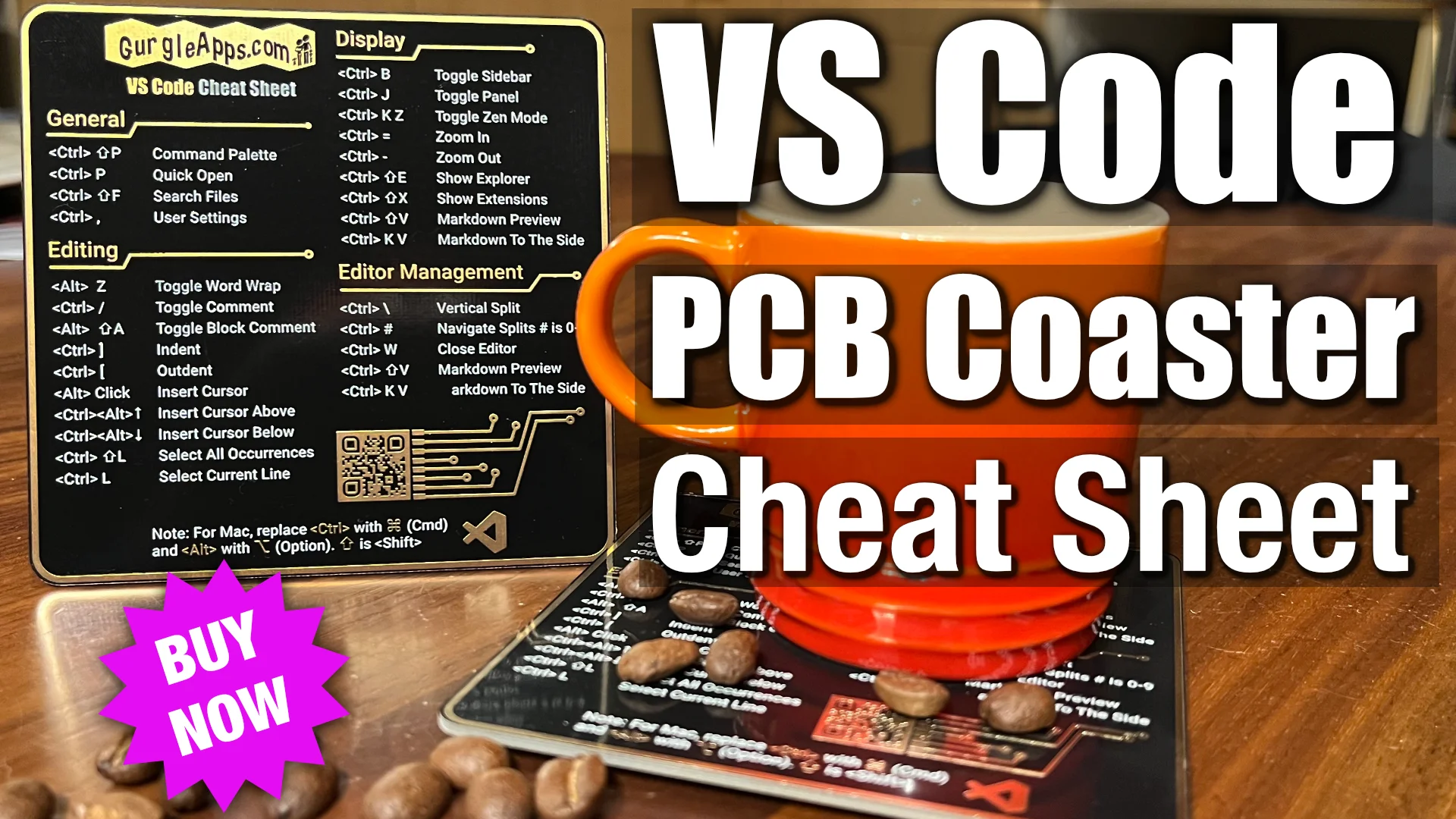